안녕하세요. 이번 포스팅에서는 웹앱 혹은 하이브리드 앱을 만들 때 사용하는 웹뷰(WebView)를 통해, 웹페이지를 어플리케이션에 보여주는 방법에 대해 알아보겠습니다 😊
웹뷰는 안드로이드 프레임워크 내부 라이브러리로 따로 implementation은 필요하지 않습니다.
인터넷 페이지를 사용하기 때문에 manifest 파일에 인터넷 권한을 요청하는 코드를 넣어주시면 됩니다.
<uses-permission android:name="android.permission.INTERNET" />
메인 액티비티에서 EditText에 URL을 입력하고, 버튼을 클릭하면 웹뷰 액티비티로 넘어가는 간단한 프로그램입니다.
그럼 코드부터 확인 하시겠습니다.
결과코드👀
<activity_main.xml>
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<EditText
android:id="@+id/enterEt"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintBottom_toBottomOf="parent"
android:text="https://tekken5953.tistory.com/" />
<Button
android:id="@+id/enterBtn"
android:layout_width="0dp"
android:layout_height="wrap_content"
app:layout_constraintStart_toStartOf="@+id/enterEt"
app:layout_constraintEnd_toEndOf="@+id/enterEt"
app:layout_constraintTop_toBottomOf="@+id/enterEt"
android:layout_marginTop="15dp"
android:text="Enter"
android:textAllCaps="false"/>
</androidx.constraintlayout.widget.ConstraintLayout>
<activity_web_view.xml>
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".WebViewActivity">
<WebView
android:id="@+id/webView"
android:layout_width="match_parent"
android:layout_height="match_parent"/>
</androidx.constraintlayout.widget.ConstraintLayout>
<MainActivity.kt>
package com.example.webviewexam
import android.content.Intent
import androidx.appcompat.app.AppCompatActivity
import android.os.Bundle
import android.widget.Button
import android.widget.EditText
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
val editText: EditText = findViewById(R.id.enterEt)
val btn: Button = findViewById(R.id.enterBtn)
val intent = Intent(this, WebViewActivity::class.java)
btn.setOnClickListener{
intent.putExtra("url",editText.text.toString())
startActivity(intent)
}
}
}
<WebViewActivity.kt>
package com.example.webviewexam
import android.annotation.SuppressLint
import android.os.Bundle
import android.util.Log
import android.webkit.WebSettings
import android.webkit.WebView
import android.webkit.WebViewClient
import androidx.appcompat.app.AppCompatActivity
class WebViewActivity : AppCompatActivity() {
@SuppressLint("SetJavaScriptEnabled")
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_web_view)
val url: String = intent.getStringExtra("url").toString()
val webView = findViewById<WebView>(R.id.webView)
val webSettings: WebSettings = webView.settings
webSettings.javaScriptEnabled = true
webSettings.loadWithOverviewMode = true
webView.webViewClient = WebViewClient()
webView.loadUrl(url)
}
}
웹뷰에 웹페이지를 띄우는 것 자체는 생각보다 간단한 것 같습니다. 웹뷰 클래스 코드에 대한 설명을 드리겠습니다.
메인 엑티비티의 EditText의 주소를 화면전환(intent) 해주는 과정에서 데이터를 담아 보내고, 웹뷰 엑티비티에서 받습니다.
intent.putExtra("url",editText.text.toString())
…
val url: String = intent.getStringExtra("url").toString()
웹뷰에서 로드하는 페이지가 자바스크립트를 사용하는 경우 자바스크립트를 허용해주어야 합니다.
webSettings.javaScriptEnabled = true
컨텐츠가 웹뷰의 크기보다 클 경우 스크린 크기에 맞게 사이즈를 자동 조정합니다.
webSettings.loadWithOverviewMode = true
새 창이 아닌 기존의 창에 덮어 씌워집니다.
webView.webViewClient = WebViewClient()
페이지를 로드합니다.
webView.loadUrl(url)
이렇게 안드로이드에서 웹뷰를 통해 웹페이지를 호출하는 방법에 대해 알아보았는데요.
다음 포스팅에서는 웹뷰에서 웹페이지를 컨트롤하는 부분에 대해 다뤄보도록 하겠습니다.
감사합니다.
+ 웹뷰에 대한 다음 포스팅 입니다.
2023.01.03 - [Android] - [안드로이드] 웹뷰(WebView)(2) - 접근과 제어
[안드로이드] 웹뷰(WebView)(2) - 접근과 제어
안녕하세요. 이전 포스팅에 이어 웹뷰에 대해 계속 알아보겠습니다. 이전엔 웹뷰를 생성하여 페이지를 로드하는 것 까지 해봤다면, 이번 글에서는 앱에서 웹뷰에 접근하고 제어하는 기능에 대
tekken5953.tistory.com
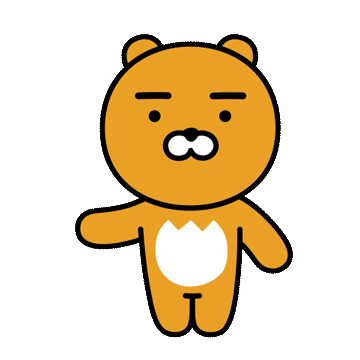
참고
https://developer.android.com/guide/webapps/webview?hl=ko#kotlin
WebView에서 웹 앱 빌드 | Android 개발자 | Android Developers
WebView에서 웹 앱 빌드 컬렉션을 사용해 정리하기 내 환경설정을 기준으로 콘텐츠를 저장하고 분류하세요. 웹 애플리케이션 또는 웹페이지만 클라이언트 애플리케이션의 일부로 제공하려는 경
developer.android.com
소스코드
https://github.com/tekken5953/WebViewExam
GitHub - tekken5953/WebViewExam
Contribute to tekken5953/WebViewExam development by creating an account on GitHub.
github.com
'Android' 카테고리의 다른 글
[안드로이드] savedInstanceState 는 왜 있는걸까? (0) | 2023.01.06 |
---|---|
[안드로이드] 웹뷰(WebView)(2) - 접근과 제어 (0) | 2023.01.03 |
[안드로이드] 아래에서 올라오는 다이얼로그 - BottomSheetDialogFragment (0) | 2022.12.14 |
[안드로이드] Spannable로 글자마다 다른 설정! (0) | 2022.12.13 |
[안드로이드] 에러 'compileDebugJavaWithJavac' task and 'compileDebugKotlin' task jvm target compatibility should be set to the same Java version. 해결 (0) | 2022.12.07 |